Hello everyone! Today’s article is the first in an extensive series introducing Blazor development to those who have never encountered it before.
Throughout this series, we’ll explore how to build modern applications using Blazor, examining the components it offers, its advantages, disadvantages, and limitations.
In this first article, we’ll cover what Blazor is, the benefits of using it, and most importantly, the foundational knowledge needed to make the most of it.
What is Blazor?
Blazor is a web frontend framework launched in 2019, based on HTML, CSS, and C#. It focuses on developing reusable components, similar to other frameworks like Angular, Vue, or React. We develop these components in Razor files, where HTML, CSS, C# (and JavaScript) come together in a single location.
C# developers will find themselves particularly comfortable developing frontend components without learning additional technologies, significantly reducing product development time.
In recent years, we’ve seen primarily client-side frameworks expanding into the backend (Node.js, anyone?). Here, we’re witnessing the opposite: a backend language integrating with client-side development.
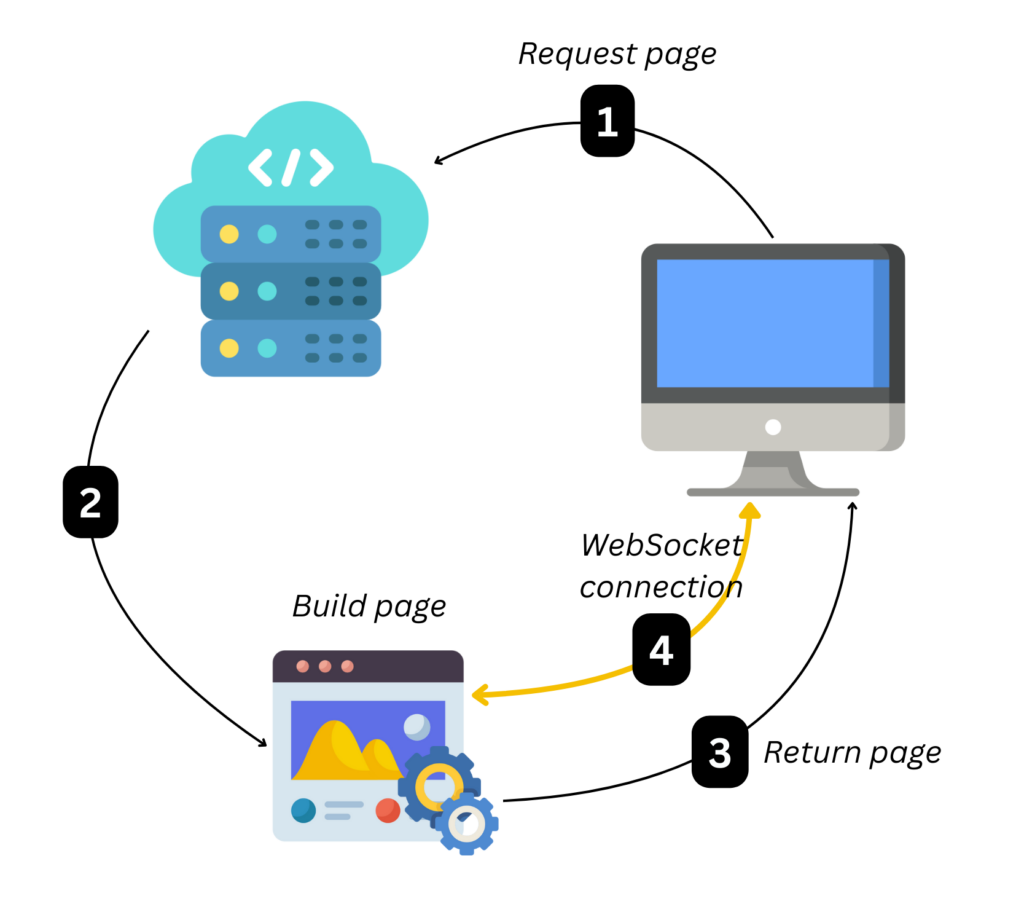
Rendering modes
Blazor offers various approaches to component rendering (organized within Razor files) and supports both client-side and server-side rendering. Let’s examine each mode in detail:
Blazor Server (server-side)
This is the default mode, where the initial page rendering occurs on the server and is then transmitted to the client. Subsequently, a WebSocket connection (SignalR) is established, and any interaction is managed by the backend (which updates its DOM image) and retransmits it to the client.
ADVANTAGES
- Very fast loading as everything is server-managed rather than client-managed
- Direct access to classes or implementations, meaning we can use dependency injection directly in our Razor views
DISADVANTAGES
- Dependence on client-server connection (every UI modification and interaction is sent to the server for processing and response)
- Suitable for small/medium-sized Single-Page Applications, as server capacity must scale with user growth
Web Assembly ( Blazor WASM)
In this mode, the .NET app is compiled and executed on the user’s client through WebAssembly.
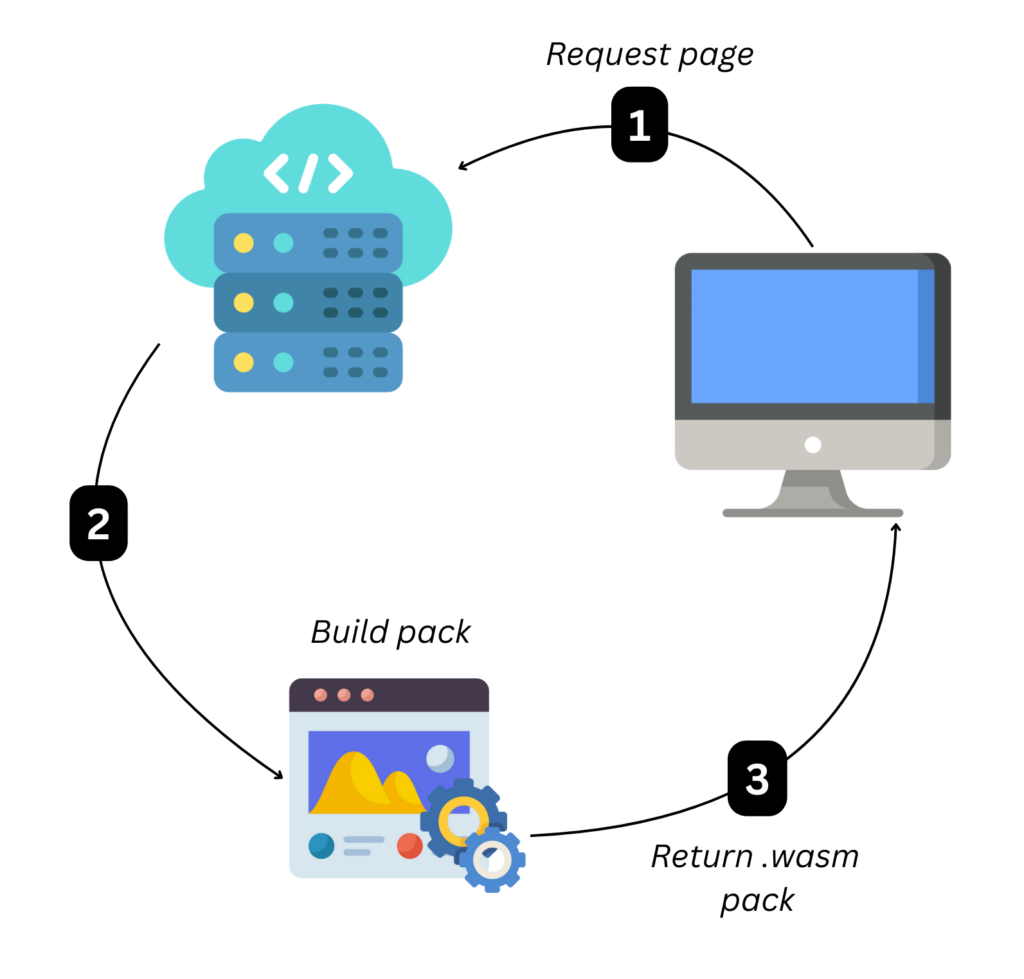
ADVANTAGES
- The webapp is connection-independent and can function offline
- Reduced server workload as processing moves to the user’s client
- Faster response time since interactions run in the browser without server dependency
- Application can be organized into component libraries (Razor Class Library), downloadable only when users interact with specific components
DISADVANTAGES
- Slower initial loading due to WebAssembly package download to the client
- More complex application architecture, requiring API endpoint implementation for resource access
InteractiveAuto
This is the newest mode, introduced with .NET 8 and enhanced in .NET 9.
Initially, the page loads using server-side mode (InteractiveServer), then downloads WebAssembly to the user’s client (if conditions allow) and continues rendering in this mode.
ADVANTAGES
- Faster initial loading
- Blazor autonomously decides whether to continue client execution or switch to server-side rendering
DISADVANTAGES
- Requires careful state management and synchronization between initial server-side rendering and subsequent client-side mode
- Only supported by modern browsers (due to WebAssembly)
Statico
As the name suggests, it loads static content without interaction. Useful for unchanging pages (e.g., privacy policy or cookie policy)
Here’s a summary of the various modes:
Mode | Interactivity | Execution | Server connection |
---|
InteractiveServer | Yes | Server | Continuous SignalR connection |
InteractiveWebAssembly | Yes | Client | None (offline possible) |
InteractiveAuto | Yes | Browser support dependent | Mixed |
Static | No | None | None |
What are the advantages and disadvantages of using Blazor?
ADVANTAGES
- Single framework for both Backend and Frontend development, enabling rapid development and project onboarding, especially for new developers or junior positions
- Dependency Injection can be used directly within Razor components (Blazor server mode only)
- Application testing in a single tool (Visual Studio), with simplified debugging
- Code can be reused across frontend and backend
DISADVANTAGES
- Blazor Web Assembly (a fully client-managed mode) isn’t supported by all browsers (including Internet Explorer!)
- Currently, Blazor Web Assembly’s performance lags behind other JavaScript frameworks
Personal Considerations
Microsoft’s new roadmap will be released in a few weeks. Major Blazor innovations came primarily with .NET 8, while .NET 9 brought minimal changes: .NET 10 could therefore hold numerous surprises for this Framework.
Microsoft rarely steps back from their products, though anything is possible. It’s worth noting that with .NET 9, they introduced .NET Aspire, and its dashboard is written in Blazor! Additionally, Blazor is used in many of Microsoft’s internal tools, suggesting (and hoping) that this framework will play a significant role in frontend development in the coming years.
Conclusions
Overall, Blazor represents an excellent choice for developers wanting to build modern interfaces without switching languages between frontend and backend. We’ve explored its strengths, available rendering modes, and main limitations, highlighting how to choose the most suitable model for your needs (server-side, WebAssembly, hybrid, or completely static).
In upcoming articles, we’ll delve deeper into each feature, learning to create reusable components, integrate business logic, and optimize performance to build complete web applications with a single .NET-based technology stack.
Happy coding with Blazor!